Form validation is a cornerstone of user-friendly web development. While HTML5 provides basic form validation, the Constraint Validation API offers advanced tools to go beyond the limitations of standard browser validation. With this API, developers gain control over custom messages, timing, error handling, and much more—all without relying on extra libraries or backend checks.
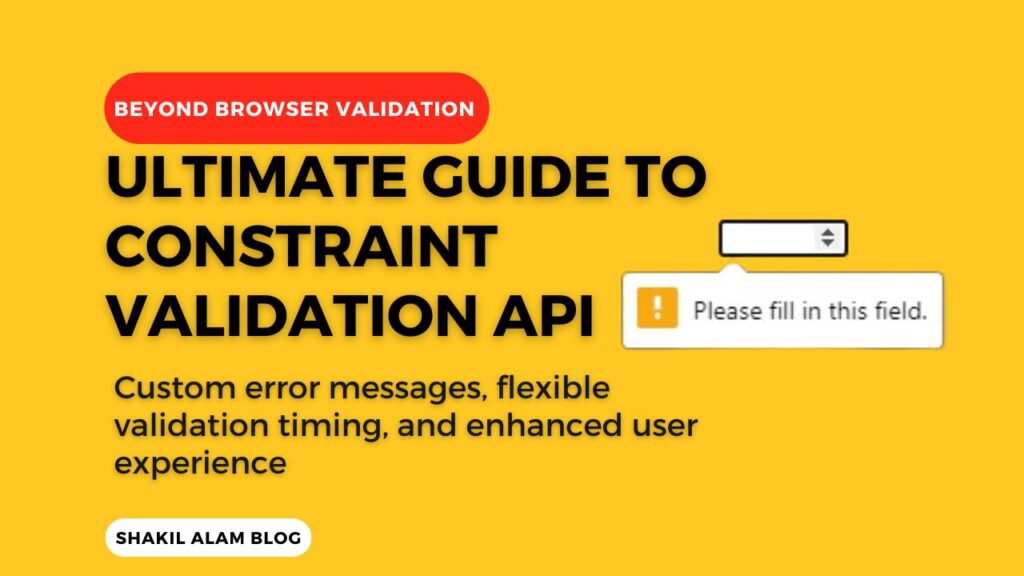
Custom error messages, flexible validation timing, and enhanced user experience
This guide covers everything you need to know to make the most of the Constraint Validation API and build forms that are robust, flexible, and user-friendly.
Table of Contents
Why Use the Constraint Validation API?
HTML5 introduced several form attributes for validation (required
, pattern
, type
, etc.), but browser validation alone can be restrictive. The messages are often generic, and the flow is automatic, making it hard to customize feedback to users.
The Constraint Validation API addresses these issues by giving you:
- Customizable Validation Feedback: Replace default error messages with specific, friendly prompts.
- Controlled Validation Timing: Validate inputs only when you want, like on blur or before submission.
- Detailed Error Identification: Pinpoint specific validation errors for a more intuitive user experience.
With the Constraint Validation API, you can enforce custom validation rules and improve the overall usability of your forms.
Key Features of the Constraint Validation API
The Constraint Validation API is packed with powerful features to control and enhance form validation. Here’s a breakdown of the core components.
1. HTML5 Validation Attributes
Even before using the API, HTML5 attributes provide a solid foundation for form validation:
required
: Ensures the field isn’t empty.type
: Controls the input format (e.g.,email
,url
).min
,max
,step
: For numeric inputs, these specify acceptable ranges and intervals.pattern
: Defines a regex pattern that the input must match.maxlength
andminlength
: Limit the length of the input.
These attributes offer a quick way to apply essential validation rules that integrate seamlessly with the Constraint Validation API.
2. Methods: checkValidity()
and reportValidity()
The API provides two main methods that are central to validation control:
checkValidity()
: Checks if all constraints are met, returningtrue
orfalse
.reportValidity()
: Not only checks validity but also displays error messages for any invalid fields.
By using these methods, you gain more control over when to validate. For instance, you can trigger validation on submission rather than letting the browser handle it automatically:
const form = document.querySelector("form");
form.addEventListener("submit", (event) => {
if (!form.checkValidity()) {
form.reportValidity();
event.preventDefault(); // Prevent submission if form is invalid
}
});
3. Custom Error Messages with setCustomValidity()
The API’s setCustomValidity()
method allows you to set custom error messages for form fields. This can be used to replace default messages with specific instructions, making it easier for users to understand what’s needed.
Example:
const emailInput = document.querySelector("input[type='email']");
emailInput.addEventListener("input", () => {
if (emailInput.validity.typeMismatch) {
emailInput.setCustomValidity("Please enter a valid email address (e.g., name@example.com).");
} else {
emailInput.setCustomValidity(""); // Clear message once valid
}
});
This method is ideal for adding personalized messages that guide users, especially if they’re entering complex data formats.
4. The validity
Property: Detailed Error Information
Every input element in a form has a validity
property, which is an object containing specific Boolean values for different validation states. With validity
, you can identify the exact reason an input is invalid.
Key validity
properties include:
valueMissing
:true
if a required field is empty.typeMismatch
:true
if the input doesn’t match the specified type.patternMismatch
:true
if the input doesn’t follow the required pattern.tooShort
andtooLong
:true
if the input length is outside the specified range.
Using validity
, you can display highly specific error messages. For example:
const usernameInput = document.querySelector("#username");
if (usernameInput.validity.tooShort) {
usernameInput.setCustomValidity("Username must be at least 5 characters long.");
} else {
usernameInput.setCustomValidity("");
}
5. The willValidate
Property
The willValidate
property is a simple Boolean that indicates whether the element will be validated when checkValidity()
or reportValidity()
is called. It’s especially useful for dynamic forms, where you may need to exclude certain fields from validation.
const inputField = document.querySelector("input");
if (inputField.willValidate) {
console.log("This field is set to be validated.");
}
Practical Benefits of the Constraint Validation API
To understand when and why to use this API over default validation, let’s look at the advantages in a table:
Feature | Default Browser Validation | Constraint Validation API |
---|---|---|
Custom Error Messages | Limited | Fully customizable with setCustomValidity() |
Validation Control | Automatic on submission | Flexible timing with checkValidity() and reportValidity() |
Detailed Error Identification | Basic and often generic | Access to validity properties for specific error reasons |
Integration with JavaScript | Limited | Full integration for custom validation flows |
Accessibility | Standard browser alerts | Tailored feedback for screen readers, customizable flow |
Best Practices for Using the Constraint Validation API
- Start with HTML5 Attributes: Use built-in attributes like
required
andtype
to establish a validation baseline. Then, layer in API features as needed. - Add
novalidate
for Custom Validations: If you’re managing validation entirely with JavaScript, add thenovalidate
attribute to the<form>
tag to disable default browser messages. - Accessible Validation: Consider using
aria-live
regions to announce validation errors for screen readers, providing an inclusive experience for all users. - Meaningful Error Messages: Avoid generic language; with
setCustomValidity()
, customize messages to be clear, actionable, and friendly. - Use Validation Timing Wisely: Avoid overwhelming users by validating too frequently. Use
checkValidity()
strategically, like on blur events, to guide them without frustration. - Friendly Error Messages: Replace technical messages with friendly, simple language. “Your email is missing ‘@’ – could you add it?” is much more helpful than “Invalid email.
Additional Reading
Constraint Validation MDN: https://developer.mozilla.org/en-US/docs/Web/HTML/Constraint_validation
Complex Constraint Example: Constraint validation – HTML: HyperText Markup Language | MDN
Client Side Form Validation: Client-side form validation – Learn web development | MDN
Constraint Validation API Browser Support (97% currently): Constraint Validation API | Can I use… Support tables for HTML5, CSS3, etc
Conclusion
The Constraint Validation API offers an advanced, flexible solution for form validation that goes far beyond the limitations of default browser validation. From custom error messages to precise error detection and validation timing, this API enables you to create forms that are robust, user-friendly, and accessible.
When you need more than basic HTML attributes to validate complex data requirements, the Constraint Validation API should be your go-to choice. Embrace its features, and you’ll soon find that validating user input can be both streamlined and, dare we say, enjoyable!
1 thought on “Mastering the Constraint Validation API: Your Ultimate Guide to Simplifying Form Validation”