I was recently redesigning my Todo App that I created 2 years ago.
When I was checking on GTmetrix, it suggested using GZIP compression for faster response times. Although I had heard of GZIP before, I had never implemented it. If you’re also in the same boat, let me introduce you to GZIP and walk you through the steps of how it works and how you can easily implement it.
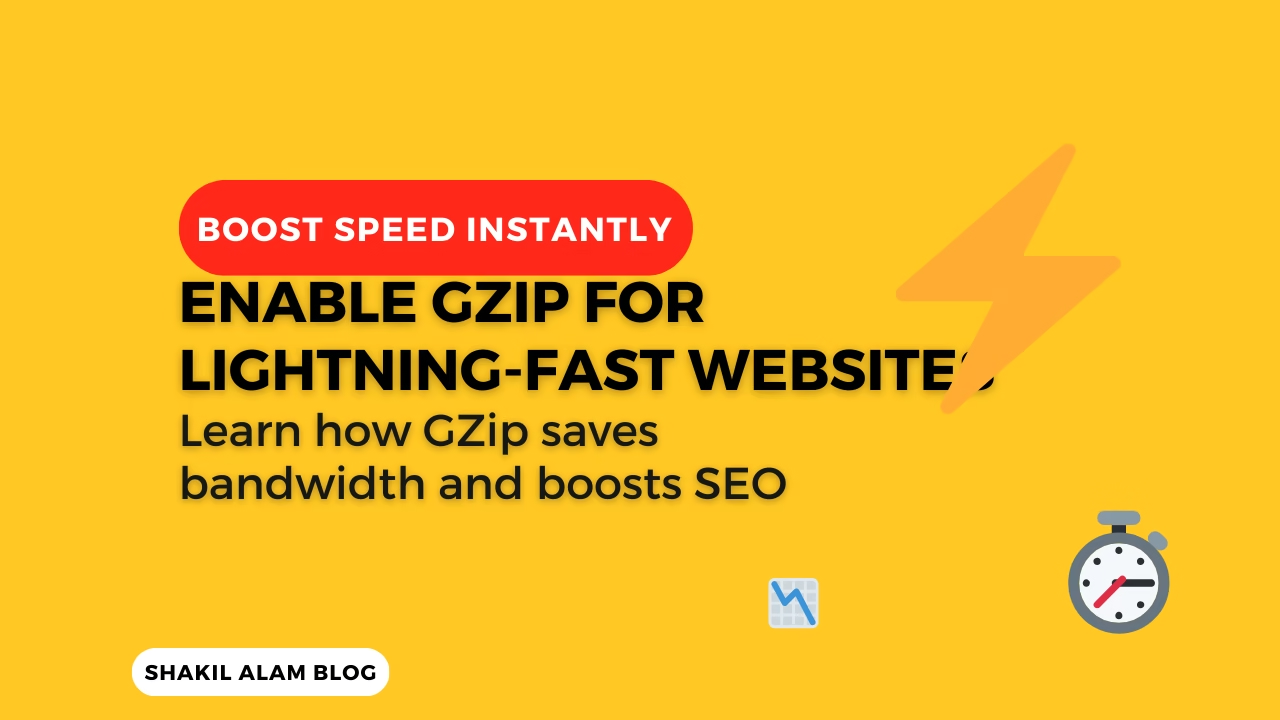
Why Use GZip?
GZip is a file compression tool that reduces the size of your web pages and assets, making them quicker to load. By compressing files such as HTML, CSS, JavaScript, and even images, you can reduce the bandwidth required to load a webpage, which leads to improved performance and a better user experience.
Here are a few reasons why you should use GZip:
- Faster Page Load Time: Smaller file sizes mean faster loading times, reducing latency and making your site feel snappy.
- Improved SEO: Google takes page load speed into account when ranking pages. Using GZip can help your site rank better in search engine results.
- Reduced Bandwidth Usage: By compressing files, the server sends less data over the network, saving bandwidth costs.
- Better User Experience: A faster website translates into happier users, reducing bounce rates and improving engagement.
How GZip Works
GZip uses a lossless compression algorithm to reduce the size of text-based files (HTML, CSS, JavaScript) by removing redundancies and unnecessary characters. When a user requests a file, the server sends the compressed version, and the browser decompresses it on the fly before rendering it. This process is transparent to the user, and all they see is a faster-loading page.
Here’s a simple breakdown of how GZip works:
- Server Compression: The web server compresses your static files (HTML, CSS, JavaScript) before sending them to the client.
- Transmission: The compressed files are sent over the network. Because they are smaller, the transmission time is reduced.
- Decompression: The client’s browser automatically decompresses the GZip files upon receiving them.
- Rendering: The browser uses the decompressed files to render the webpage as usual.
GZip compression typically reduces the size of text-based files by around 60-80%. For example, a 200KB JavaScript file can be compressed down to 50KB, resulting in faster loading times and lower bandwidth consumption.
How to Implement GZip
Implementing GZip compression is straightforward and can be done in a few steps, depending on your server setup. Here’s how you can enable GZip on popular web servers:
1. For Apache Servers
If you’re using Apache, GZip compression is simple to enable via the .htaccess
file.
Add the following code to your .htaccess
file in the root directory of your site:
# Enable GZip compression
<IfModule mod_deflate.c>
# Compress HTML, CSS, JavaScript, and more
AddOutputFilterByType DEFLATE text/html text/plain text/xml text/css application/javascript application/json
# Ensure that already compressed files are not re-compressed
SetEnvIf Request_URI \.(?:gif|jpe?g|png|pdf)$ no-gzip dont-vary
</IfModule>
2. For Nginx Servers
If you’re using Nginx, you’ll need to modify your server configuration to enable GZip.
Open your nginx.conf
file and add the following lines inside the http
block:
# Enable GZip compression
gzip on;
gzip_types text/plain text/css application/javascript application/json text/xml application/xml application/xml+rss text/javascript;
gzip_min_length 1000;
gzip_proxied any;
gzip_vary on;
This will enable GZip compression for various content types, including plain text, CSS, JavaScript, and JSON.
3. For Node.js Servers
If you’re using Node.js with Express, you can enable GZip compression using the compression
middleware.
First, install the compression package:
npm install compression
Then, in your server.js
or main application file, add the following:
const express = require('express');
const compression = require('compression');
const app = express();
// Use GZip compression for all responses
app.use(compression());
app.get('/', (req, res) => {
res.send('Hello, GZip!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
4. For Other Web Servers or CMS
For other setups, such as hosting on platforms like WordPress, you can either configure GZip through your web host’s control panel or use plugins designed to enable compression.
For WordPress, the WP Super Cache plugin or W3 Total Cache plugin offers built-in GZip support, and you can activate it via the plugin settings.
5. Verify GZip Compression
Once you’ve implemented GZip, it’s essential to verify that it’s working. You can use tools like GTmetrix, Pingdom, or WebPageTest to check if your files are being served with GZip compression. Additionally, you can inspect the response headers in the browser’s Developer Tools (Network tab) and look for the Content-Encoding: gzip
header.
Conclusion
Implementing GZip compression is one of the easiest and most effective ways to optimize your website’s performance. By reducing the size of your files, you can drastically improve load times, reduce bandwidth usage, and enhance user experience.
Don’t wait—enable GZip today and start seeing improvements in your site’s speed and efficiency!
Suggested Reading: 3 GitHub Hacks You Didn’t Know You Needed (But Totally Do!) 🚀