If you’re tired of manually running migrations, clearing caches, and recaching configurations every time you git pull
or git merge
, Git hooks are here to make your life easier. But rather than running every command every time, let’s make this hook smarter, so it only performs these actions when necessary.
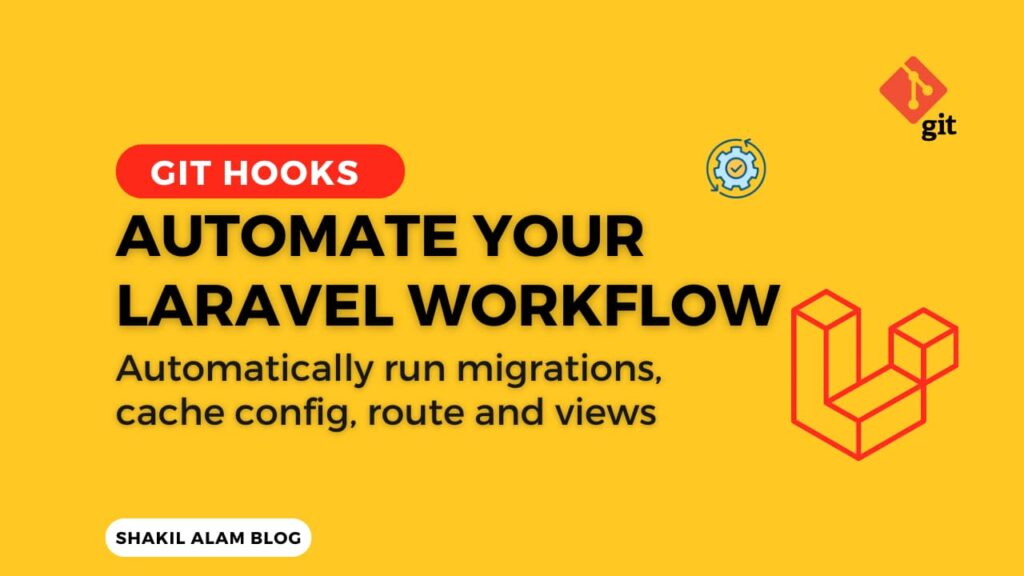
This post will walk you through setting up a post-merge hook that checks for changes in specific files or folders and only runs migrations, recaches configuration, views, and routes if something’s been updated. Let’s dive in!
Table of Contents
What Are Git Hooks?
Git hooks are scripts that Git runs before or after specific events, such as committing, merging, or checking out code. For our setup, we’ll use the post-merge hook, which runs every time you pull or merge new changes into your local repository. This way, your development environment will always be ready to go without you lifting a finger.
You can find all available Git hooks in the .git/hooks
directory of your repository. Hooks are typically simple Bash scripts, and by customizing them, you can automate almost any task you need.
Setting Up a Smart Post-Merge Hook
Let’s set up a post-merge hook that will:
- Check if there are new migration files and run php artisan migrate only if necessary.
- Recache configuration, views, and routes only if those files have changed.
- Locate or Create the
post-merge
File. Navigate to the.git/hooks
directory in your project. If you don’t already see apost-merge
file, create one there. - Write the Script with Conditions for Each Action. Here’s a smart, optimized script that checks for changes before running commands:
#!/bin/bash
echo "Running optimized post-merge hook..."
# Check for new migration files
if git diff --name-only HEAD@{1} HEAD | grep -qE '^database/migrations/.*\.php'; then
echo "New migrations found. Running database migrations..."
php artisan migrate --force
else
echo "No new migrations found. Skipping migration step."
fi
# Check for changes in config files
if git diff --name-only HEAD@{1} HEAD | grep -qE '^config/.*\.php'; then
echo "Config changes detected. Recaching config..."
php artisan config:cache
else
echo "No config changes detected. Skipping config cache."
fi
# Check for changes in view files
if git diff --name-only HEAD@{1} HEAD | grep -qE '^resources/views/.*\.(php|blade\.php)'; then
echo "View changes detected. Recaching views..."
php artisan view:cache
else
echo "No view changes detected. Skipping view cache."
fi
# Check for changes in route files
if git diff --name-only HEAD@{1} HEAD | grep -qE '^routes/.*\.php'; then
echo "Route changes detected. Recaching routes..."
php artisan route:cache
else
echo "No route changes detected. Skipping route cache."
fi
echo "Optimized post-merge tasks completed!"
Explanation:
- Database Migrations: Checks if there are new migration files in
database/migrations
. If found, it runsphp artisan migrate --force
to apply changes automatically. - Config Caching: Checks for changes in
config
files and recaches if needed. - View Caching: Looks for updates in
resources/views
to decide whether view caching is necessary. - Route Caching: Checks if any files in the
routes
directory have been modified, and recaches if they have.
3. Make Your Script Executable. Run the following command to allow Git to execute the hook:
chmod +x .git/hooks/post-merge
- Test It Out! Now, whenever you pull or merge changes with updated migration, config, views, or route files, only the necessary tasks will run. This keeps your development environment synced and responsive, without unnecessary processing.
Now, whenever you pull or merge changes with updated migration, config, views, or route files, only the necessary tasks will run. This keeps your development environment synced and responsive, without unnecessary processing.
You can even find the code at https://gist.github.com/itxshakil/54845e124959bfb70d040964ba0a0eef
Error Handling and Debugging the Script
While the script is designed to run automatically, errors can still occur. Here are some troubleshooting tips:
- Permissions Issues: Ensure that the post-merge file has executable permissions. If you encounter errors, run
chmod +x .git/hooks/post-merge
again. - Failed Migrations: If a migration fails, the script will not prevent further commands from executing. To avoid this, you could add error handling for the php artisan migrate command to stop the script if an error occurs:
php artisan migrate --force || { echo "Migration failed. Exiting."; exit 1; }
- Debugging: You can add debug lines to log output and errors to a file for easier tracking: bash
echo "Starting migration check…" >> migration.log
- Check Hook Execution: If the script is not being executed, make sure that the
.git/hooks
directory is not being ignored by Git (.gitignore should not include this folder).
By adding logging and error handling, you’ll be able to track any issues that arise more effectively.
Key Takeaways
- Automate Smartly: By adding conditions, this Git hook only runs migrations, config caching, view caching, or route caching when needed, saving time and resources.
- Easy to Implement: Just a few lines of Bash scripting streamline your setup tasks automatically.
- Keep Your Environment in Sync with Minimal Effort: This hook ensures that your local environment reflects the latest changes without manual intervention.
- Error Handling & Debugging: Implementing logging and error handling improves the script’s reliability, especially in larger projects.
Mastering Laravel’s phpunit.xml: An In-Depth Guide
Conclusion
Setting up this optimized Git hook is a game-changer, especially for projects with frequent updates. With just a few lines of code, you get a fast, automated, and up-to-date workflow—no more missed migrations or forgotten caches. Try it out, and let Git take care of the busy work for you!
Bonus: For more advanced Git hook setups or if you need additional hooks for other events, check out the full Git Hooks documentation.
Happy coding, and here’s to a smoother, smarter development experience!
3 COMMENTS